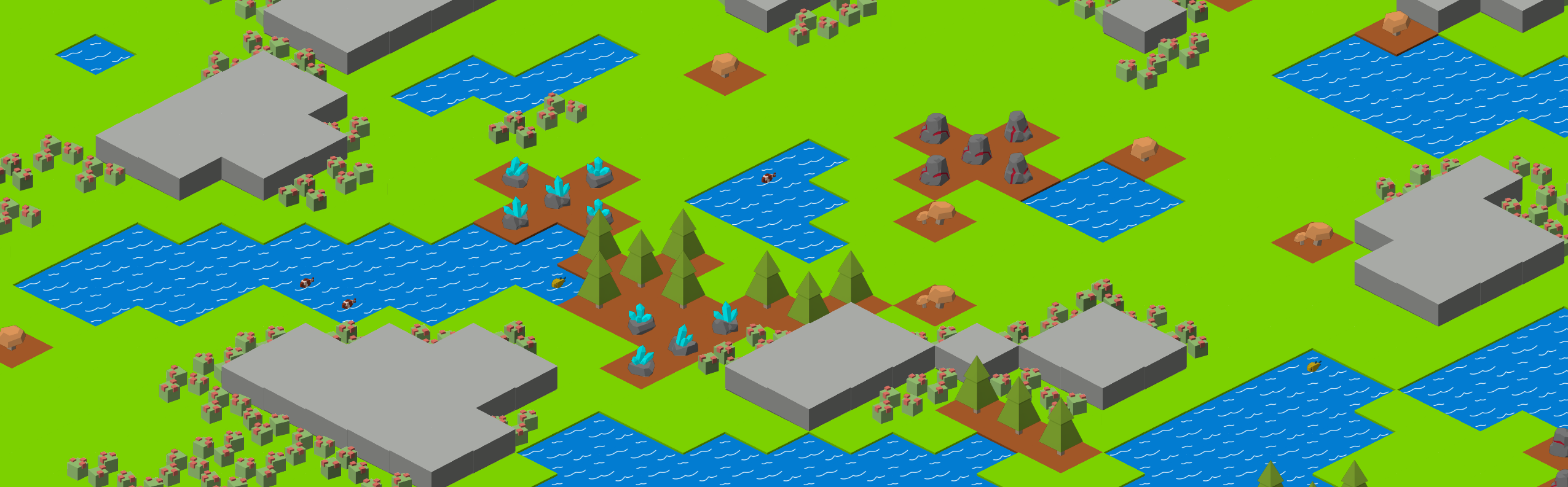
Neural MMO provides a pettingzoo compliant environment API, an extensive configuration system, and support for agent scripting, OpenSkill evaluation, custom terrain generation, and overlay visualization. This section is intended as a reference – the tutorials provide a much better starting point for most users.
Environment#
- class nmmo.Env(config: ~nmmo.core.config.Default = <nmmo.core.config.Default object>, seed=None)
- action_space(agent)
Neural MMO Action Space
- Args:
agent: Agent ID
- Returns:
actions: gym.spaces object contained the structured actions for the specified agent. Each action is parameterized by a list of discrete-valued arguments. These consist of both fixed, k-way choices (such as movement direction) and selections from the observation space (such as targeting)
- property agents: List[str]
For conformity with the PettingZoo API only; rendering is external
- change_task(new_tasks: List[Union[Tuple[Task, float], Task]], task_encoding: Optional[Dict[int, ndarray]] = None, embedding_size: int = 16, reset: bool = True, map_id=None, seed=None, options=None)
Changes the task given to each agent
- Args:
new_task: The task to complete and calculate rewards task_encoding: A mapping from eid to encoded task embedding_size: The size of each embedding reset: Resets the environment
- close()
For conformity with the PettingZoo API only; rendering is external
- observation_space(agent: int)
Neural MMO Observation Space
- Args:
agent: Agent ID
- Returns:
observation: gym.spaces object contained the structured observation for the specified agent. Each visible object is represented by continuous and discrete vectors of attributes. A 2-layer attentional encoder can be used to convert this structured observation into a flat vector embedding.
- render(mode='human')
For conformity with the PettingZoo API only; rendering is external
- reset(map_id=None, seed=None, options=None)
OpenAI Gym API reset function
Loads a new game map and returns initial observations
- Args:
idx: Map index to load. Selects a random map by default
- Returns:
observations, as documented by _compute_observations()
- Notes:
Neural MMO simulates a persistent world. Ideally, you should reset the environment only once, upon creation. In practice, this approach limits the number of parallel environment simulations to the number of CPU cores available. At small and medium hardware scale, we therefore recommend the standard approach of resetting after a long but finite horizon: ~1000 timesteps for small maps and 5000+ timesteps for large maps
- seed(seed=None)
Reseeds the environment (making it deterministic).
- state() ndarray
State returns a global view of the environment appropriate for centralized training decentralized execution methods like QMIX
- step(actions: Dict[int, Dict[str, Dict[str, Any]]])
Simulates one game tick or timestep
- Args:
actions: A dictionary of agent decisions of format:
{ agent_1: { action_1: [arg_1, arg_2], action_2: [...], ... }, agent_2: { ... }, ... } Where agent_i is the integer index of the i'th agent The environment only evaluates provided actions for provided gents. Unprovided action types are interpreted as no-ops and illegal actions are ignored It is also possible to specify invalid combinations of valid actions, such as two movements or two attacks. In this case, one will be selected arbitrarily from each incompatible sets. A well-formed algorithm should do none of the above. We only Perform this conditional processing to make batched action computation easier.
- Returns:
(dict, dict, dict, None):
- observations:
A dictionary of agent observations of format:
{ agent_1: obs_1, agent_2: obs_2, ... }
Where agent_i is the integer index of the i’th agent and obs_i is specified by the observation_space function.
- rewards:
A dictionary of agent rewards of format:
{ agent_1: reward_1, agent_2: reward_2, ... }
Where agent_i is the integer index of the i’th agent and reward_i is the reward of the i’th’ agent.
By default, agents receive -1 reward for dying and 0 reward for all other circumstances. Override Env.reward to specify custom reward functions
- dones:
A dictionary of agent done booleans of format:
{ agent_1: done_1, agent_2: done_2, ... }
Where agent_i is the integer index of the i’th agent and done_i is a boolean denoting whether the i’th agent has died.
Note that obs_i will be a garbage placeholder if done_i is true. This is provided only for conformity with PettingZoo. Your algorithm should not attempt to leverage observations outside of trajectory bounds. You can omit garbage obs_i values by setting omitDead=True.
- infos:
A dictionary of agent infos of format:
- {
agent_1: None, agent_2: None, …
}
Provided for conformity with PettingZoo
- class nmmo.Agent(config, idx)
- __call__(obs)
Used by scripted agents to compute actions. Override in subclasses.
- Args:
obs: Agent observation provided by the environment
- __init__(config, idx)
Base class for agents
- Args:
config: A Config object idx: Unique AgentID int
Config#
- class nmmo.config.Combat
Combat Game System
- COMBAT_DAMAGE_FORMULA(offense, defense, multiplier)
Damage formula
- COMBAT_MAGE_DAMAGE = 30
Mage attack damage
- COMBAT_MAGE_REACH = 3
Reach of attacks using the Mage skill
- COMBAT_MELEE_DAMAGE = 30
Melee attack damage
- COMBAT_MELEE_REACH = 3
Reach of attacks using the Melee skill
- COMBAT_RANGE_DAMAGE = 30
Range attack damage
- COMBAT_RANGE_REACH = 3
Reach of attacks using the Range skill
- COMBAT_SPAWN_IMMUNITY = 20
Agents older than this many ticks cannot attack agents younger than this many ticks
- COMBAT_STATUS_DURATION = 3
Combat status lasts for this many ticks after the last combat event. Combat events include both attacking and being attacked.
- COMBAT_SYSTEM_ENABLED = True
Game system flag
- COMBAT_WEAKNESS_MULTIPLIER = 1.5
Multiplier for super-effective attacks
- class nmmo.config.Config
An environment configuration object
Global constants are defined as static class variables. You can override any Config variable using standard CLI syntax (e.g. –NENT=128).
The default config as of v1.5 uses 1024x1024 maps with up to 2048 agents and 1024 NPCs. It is suitable to time horizons of 8192+ steps. For smaller experiments, consider the SmallMaps config.
- Notes:
We use Google Fire internally to replace standard manual argparse definitions for each Config property. This means you can subclass Config to add new static attributes – CLI definitions will be generated automatically.
- BASE_HEALTH = 10
Initial Constitution level and agent health
- EMULATE_CONST_HORIZON = False
Emulate a constant HORIZON simulations steps
- EMULATE_CONST_PLAYER_N = False
Emulate a constant number of agents
- EMULATE_FLAT_ATN = False
Emulate a flat action space
- EMULATE_FLAT_OBS = False
Emulate a flat observation space
- IMMORTAL = False
Debug parameter: prevents agents from dying except by void
- LOG_ENV = False
Whether to log env steps (expensive)
- LOG_EVENTS = True
Whether to log events (semi-expensive)
- LOG_FILE = None
Where to write logs (defaults to console)
- LOG_MILESTONES = True
Whether to log server-firsts (semi-expensive)
- LOG_VERBOSE = False
Whether to log server messages or just stats
- MAP_BORDER = 16
Number of void border tiles surrounding each side of the map
- MAP_CENTER = None
Size of each map (number of tiles along each side)
- MAP_FORCE_GENERATION = True
Whether to regenerate and overwrite existing maps
- MAP_GENERATE_PREVIEWS = False
Whether map generation should also save .png previews (slow + large file size)
- MAP_GENERATOR
alias of
MapGenerator
- MAP_N = 1
Number of maps to generate
- property MAP_N_OBS
Number of distinct tile observations
- MAP_N_TILE = 16
Number of distinct terrain tile types
- MAP_PREVIEW_DOWNSCALE = 1
Downscaling factor for png previews
- property MAP_SIZE
- PATH_CWD = '/workspaces/PufferTank/nmmo-beta-docs'
Working directory
- PATH_MAPS = None
Generated map directory
- PATH_MAP_SUFFIX = 'map{}/map.npy'
Map file name
- PATH_RESOURCE = '/workspace/nmmo-environment/nmmo/resource'
Resource directory
- PATH_ROOT = '/workspace/nmmo-environment/nmmo'
Global repository directory
- PATH_TILE = '/workspace/nmmo-environment/nmmo/resource/{}.png'
Tile path – format me with tile name
- PLAYERS = [<class 'nmmo.core.agent.Agent'>]
Player classes from which to spawn
- PLAYER_BASE_HEALTH = 100
Initial agent health
- PLAYER_DEATH_FOG = None
How long before spawning death fog. None for no death fog
- PLAYER_DEATH_FOG_FINAL_SIZE = 8
Number of tiles from the center that the fog stops
- PLAYER_DEATH_FOG_SPEED = 1
Number of tiles per tick that the fog moves in
- PLAYER_LOADER
alias of
SequentialLoader
- PLAYER_N = None
Maximum number of players spawnable in the environment
- PLAYER_N_OBS = 100
Number of distinct agent observations
- property PLAYER_POLICIES
Number of player policies
- PLAYER_SPAWN_TEAMMATE_DISTANCE = 1
Buffer tiles between teammates at spawn
- property PLAYER_TEAM_SIZE
- property PLAYER_VISION_DIAMETER
Size of the square tile crop visible to an agent
- PLAYER_VISION_RADIUS = 7
Number of tiles an agent can see in any direction
- PROVIDE_ACTION_TARGETS = False
Flag used to provide action targets mask
- game_system_enabled(name) bool
- class nmmo.config.Large
A large config suitable for large-scale research or fast models
- HORIZON = 8192
- MAP_CENTER = 1024
Size of each map (number of tiles along each side)
- MAP_PREVIEW_DOWNSCALE = 64
Downscaling factor for png previews
- NPC_LEVEL_MAX = 15
- NPC_LEVEL_SPREAD = 3
- NPC_N = 1024
- PATH_MAPS = 'maps/large'
Generated map directory
- PLAYER_N = 1024
Maximum number of players spawnable in the environment
- PROGRESSION_SPAWN_CLUSTERS = 1024
- PROGRESSION_SPAWN_UNIFORMS = 4096
- class nmmo.config.Medium
A medium config suitable for most academic-scale research
- HORIZON = 1024
- MAP_CENTER = 128
Size of each map (number of tiles along each side)
- MAP_PREVIEW_DOWNSCALE = 16
Downscaling factor for png previews
- NPC_LEVEL_MAX = 10
- NPC_LEVEL_SPREAD = 1
- NPC_N = 128
- PATH_MAPS = 'maps/medium'
Generated map directory
- PLAYER_N = 128
Maximum number of players spawnable in the environment
- PROGRESSION_SPAWN_CLUSTERS = 64
- PROGRESSION_SPAWN_UNIFORMS = 256
- class nmmo.config.NPC
NPC Game System
- NPC_BASE_DAMAGE = 15
Base NPC damage
- NPC_BASE_DEFENSE = 0
Base NPC defense
- NPC_LEVEL_DAMAGE = 30
Bonus NPC damage per level
- NPC_LEVEL_DEFENSE = 30
Bonus NPC defense per level
- NPC_LEVEL_MAX = 10
Maximum NPC level
- NPC_LEVEL_MIN = 1
Minimum NPC level
- NPC_N = None
Maximum number of NPCs spawnable in the environment
- NPC_SPAWN_AGGRESSIVE = 0.8
Percentage distance threshold from spawn for aggressive NPCs
- NPC_SPAWN_ATTEMPTS = 25
Number of NPC spawn attempts per tick
- NPC_SPAWN_NEUTRAL = 0.5
Percentage distance threshold from spawn for neutral NPCs
- NPC_SPAWN_PASSIVE = 0.0
Percentage distance threshold from spawn for passive NPCs
- NPC_SYSTEM_ENABLED = True
Game system flag
- class nmmo.config.Progression
Progression Game System
- PROGRESSION_AMMUNITION_XP_SCALE = 1
Multiplier on top of XP_SCALE for Prospecting, Carving, and Alchemy
- PROGRESSION_BASE_DEFENSE = 0
Base defense
- PROGRESSION_BASE_LEVEL = 1
Initial skill level
- PROGRESSION_BASE_XP_SCALE = 1
Base XP awarded for each skill usage – multiplied by skill level
- PROGRESSION_COMBAT_XP_SCALE = 1
Multiplier on top of XP_SCALE for Melee, Range, and Mage
- PROGRESSION_CONSUMABLE_XP_SCALE = 5
Multiplier on top of XP_SCALE for Fishing and Herbalism
- PROGRESSION_LEVEL_DEFENSE = 5
Bonus defense per level
- PROGRESSION_LEVEL_MAX = 10
Max skill level
- PROGRESSION_MAGE_BASE_DAMAGE = 0
Base Mage attack damage
- PROGRESSION_MAGE_LEVEL_DAMAGE = 5
Bonus Mage attack damage per level
- PROGRESSION_MELEE_BASE_DAMAGE = 0
Base Melee attack damage
- PROGRESSION_MELEE_LEVEL_DAMAGE = 5
Bonus Melee attack damage per level
- PROGRESSION_RANGE_BASE_DAMAGE = 0
Base Range attack damage
- PROGRESSION_RANGE_LEVEL_DAMAGE = 5
Bonus Range attack damage per level
- PROGRESSION_SYSTEM_ENABLED = True
Game system flag
- class nmmo.config.Resource
Resource Game System
- RESOURCE_BASE = 100
Initial level and capacity for food and water
- RESOURCE_DEHYDRATION_RATE = 10
Damage per tick without water
- RESOURCE_DEPLETION_RATE = 5
Depletion rate for food and water
- RESOURCE_FOILAGE_CAPACITY = 1
Maximum number of harvests before a foilage tile decays
- RESOURCE_FOILAGE_RESPAWN = 0.025
Probability that a harvested foilage tile will regenerate each tick
- RESOURCE_HARVEST_RESTORE_FRACTION = 1.0
Fraction of maximum capacity restored upon collecting a resource
- RESOURCE_HEALTH_REGEN_THRESHOLD = 0.5
Fraction of maximum resource capacity required to regen health
- RESOURCE_HEALTH_RESTORE_FRACTION = 0.1
Fraction of health restored per tick when above half food+water
- RESOURCE_STARVATION_RATE = 10
Damage per tick without food
- RESOURCE_SYSTEM_ENABLED = True
Game system flag
- class nmmo.config.Small
A small config for debugging and experiments with an expensive outer loop
- HORIZON = 128
- MAP_CENTER = 32
Size of each map (number of tiles along each side)
- MAP_PREVIEW_DOWNSCALE = 4
Downscaling factor for png previews
- NPC_LEVEL_MAX = 5
- NPC_LEVEL_SPREAD = 1
- NPC_N = 32
- PATH_MAPS = 'maps/small'
Generated map directory
- PLAYER_N = 64
Maximum number of players spawnable in the environment
- PROGRESSION_SPAWN_CLUSTERS = 4
- PROGRESSION_SPAWN_UNIFORMS = 16
- TERRAIN_LOG_INTERPOLATE_MIN = 0
Procedural Generation#
The default map generator is multioctave perlin noise that is itself seeded using perlin noise to create terrain of varying local frequency. Use the MAP_GENERATOR config argument to supply your own generator classes. We suggest subclassing the default and overriding the generate_map method. For procedural generation beyond maps, customize game system mechanics dynamically to modify mechanics per-environment.
- class nmmo.MapGenerator(config)
Procedural map generation
- generate_all_maps()
Generates NMAPS maps according to generate_map
Provides additional utilities for saving to .npy and rendering png previews
- generate_map(idx)
Generate a single map
The default method is a relatively complex multiscale perlin noise method. This is not just standard multioctave noise – we are seeding multioctave noise itself with perlin noise to create localized deviations in scale, plus additional biasing to decrease terrain frequency towards the center of the map
We found that this creates more visually interesting terrain and more deviation in required planning horizon across different parts of the map. This is by no means a gold-standard: you are free to override this method and create customized terrain generation more suitable for your application. Simply pass MAP_GENERATOR=YourMapGenClass as a config argument.
- load_textures()
Called during setup; loads and resizes tile pngs
- class nmmo.Terrain
Terrain material class; populated at runtime